Advanced Telegram Desktop Tricks with Telethon: Automating Tasks on Your PC
Telethon is a powerful Python library that allows you to interact with Telegram’s API—enabling you to automate tasks, manage chats, and build custom tools. In this tutorial, we’ll guide you through setting up Telethon on your PC, walk you through some basic and advanced automation scripts, and discuss best practices for secure, efficient automation.
1. Setting Up Your Environment
Before you start automating tasks with Telethon, you'll need to prepare your environment:
- Install Python: Ensure Python 3.6 or higher is installed. Download it from the official Python website.
- Install Telethon: Open your command prompt or terminal and run:
pip install telethon
- Obtain API Credentials: Log in at my.telegram.org to create a new application and obtain your API ID and API Hash.
After setting up your environment, you’re ready to start coding.
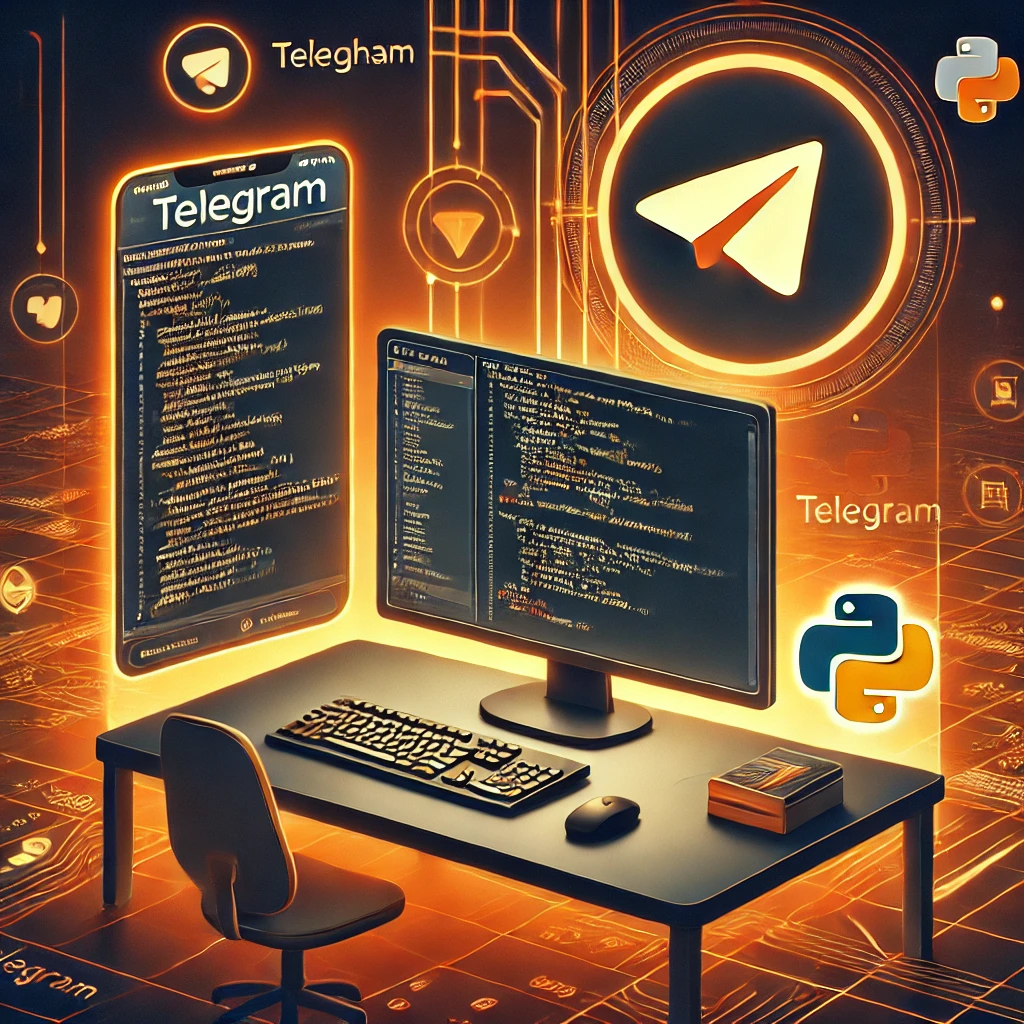
2. Your First Telethon Script: Logging In and Sending a Message
Let’s write a simple script that logs you in and sends a message to yourself:
from telethon import TelegramClient, events
# Replace with your own values
api_id = YOUR_API_ID
api_hash = 'YOUR_API_HASH'
phone = 'YOUR_PHONE_NUMBER'
# Create the client and connect
client = TelegramClient('session_name', api_id, api_hash)
async def main():
await client.start(phone)
print("Logged in successfully!")
await client.send_message('me', 'Hello from Telethon!')
with client:
client.loop.run_until_complete(main())
This script creates a session, logs into your Telegram account, and sends a message to yourself. Customize the code as needed for your automation tasks.
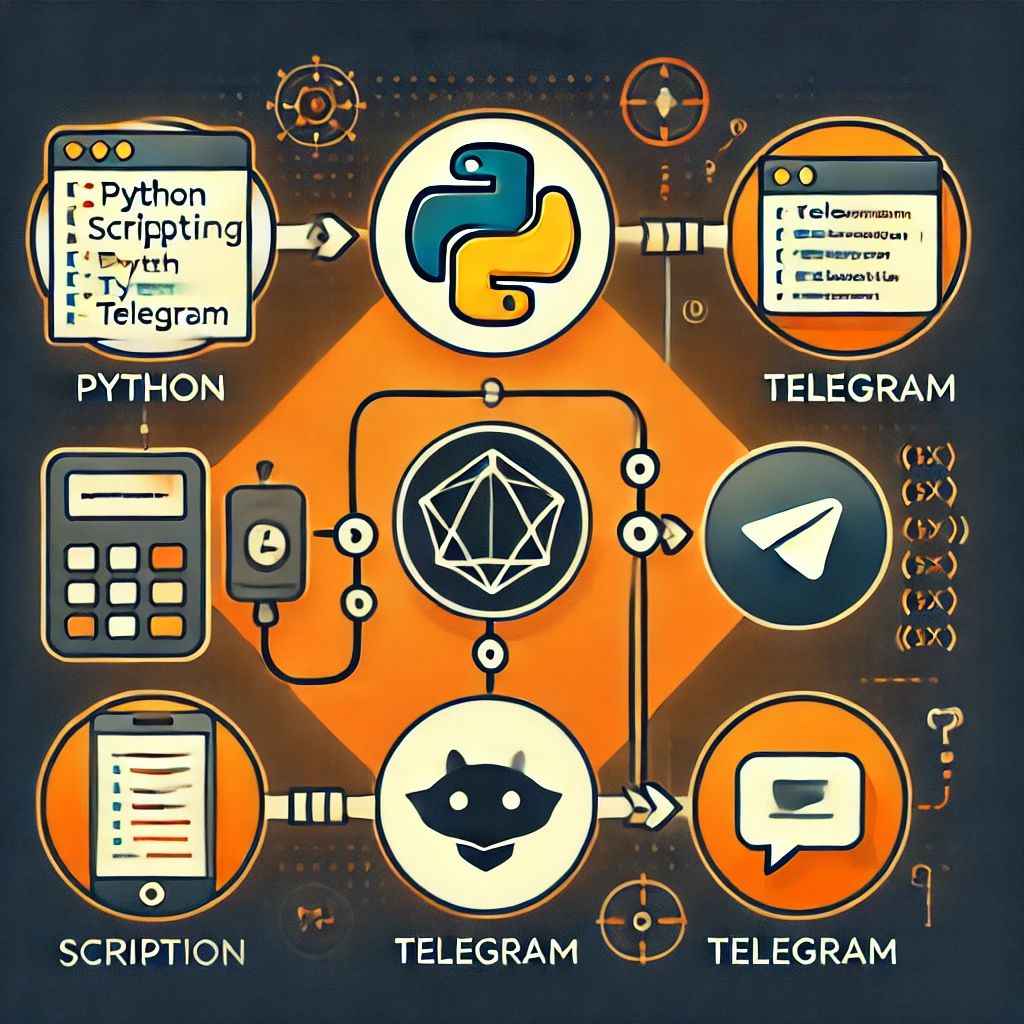
3. Advanced Automation Tasks with Telethon
Once you’re comfortable with the basics, you can explore more advanced automation tasks. Here are a few examples:
- Auto-Forwarding Messages: Write a script that listens for new messages in a specific chat and automatically forwards them to another chat.
- Scheduled Messaging: Use Python's
asyncio
library to schedule messages at specific times, perfect for reminders or announcements. - Bulk Message Deletion: Automate the cleanup of old messages or spam by filtering and deleting messages based on date or content.
Below is an example of an auto-forwarding script:
from telethon import TelegramClient, events
api_id = YOUR_API_ID
api_hash = 'YOUR_API_HASH'
client = TelegramClient('session_name', api_id, api_hash)
@client.on(events.NewMessage(chats='source_chat'))
async def handler(event):
await client.forward_messages('target_chat', event.message)
with client:
client.run_until_disconnected()
Experiment with these advanced tasks to streamline your workflow and improve productivity.
4. Best Practices and Security Considerations
When automating tasks with Telethon, keep these best practices in mind:
- Protect Your API Credentials: Never expose your API ID and hash in public repositories.
- Respect Rate Limits: Avoid overloading Telegram’s servers by implementing delays and proper error handling.
- Test in a Controlled Environment: Run your scripts in a sandbox before deploying them broadly.
- Monitor Logs: Regularly review logs to catch any unexpected behavior or errors early.
Conclusion
Telethon opens up a world of possibilities for automating your Telegram desktop experience. Whether you’re scheduling messages, auto-forwarding important updates, or managing groups and channels more efficiently, these advanced tricks can save you time and boost productivity.
By following this tutorial and experimenting with various scripts, you can unlock the full potential of Telegram automation on your PC. Happy coding and automating!
0 Comments
Leave a Comment