How to Build a Cryptocurrency Price Alert Bot on Telegram Using Python: A Step-by-Step Tutorial
This tutorial will guide you through creating a cryptocurrency price alert bot on Telegram using Python. With real-time data from a cryptocurrency API, your bot will notify you when prices cross certain thresholds. Whether you're a crypto enthusiast or a trader, this bot can help you stay informed and make timely decisions.
1. Prerequisites and Setup
Before we start, ensure you have the following:
- Python 3.6 or higher installed on your PC.
- Basic knowledge of Python programming.
- An API key from a cryptocurrency data provider (e.g., CoinGecko API is free and reliable).
- A Telegram account and a bot created using
@BotFather
.
Install the necessary Python libraries by running:
pip install python-telegram-bot requests
Once you have everything set up, you're ready to start coding.
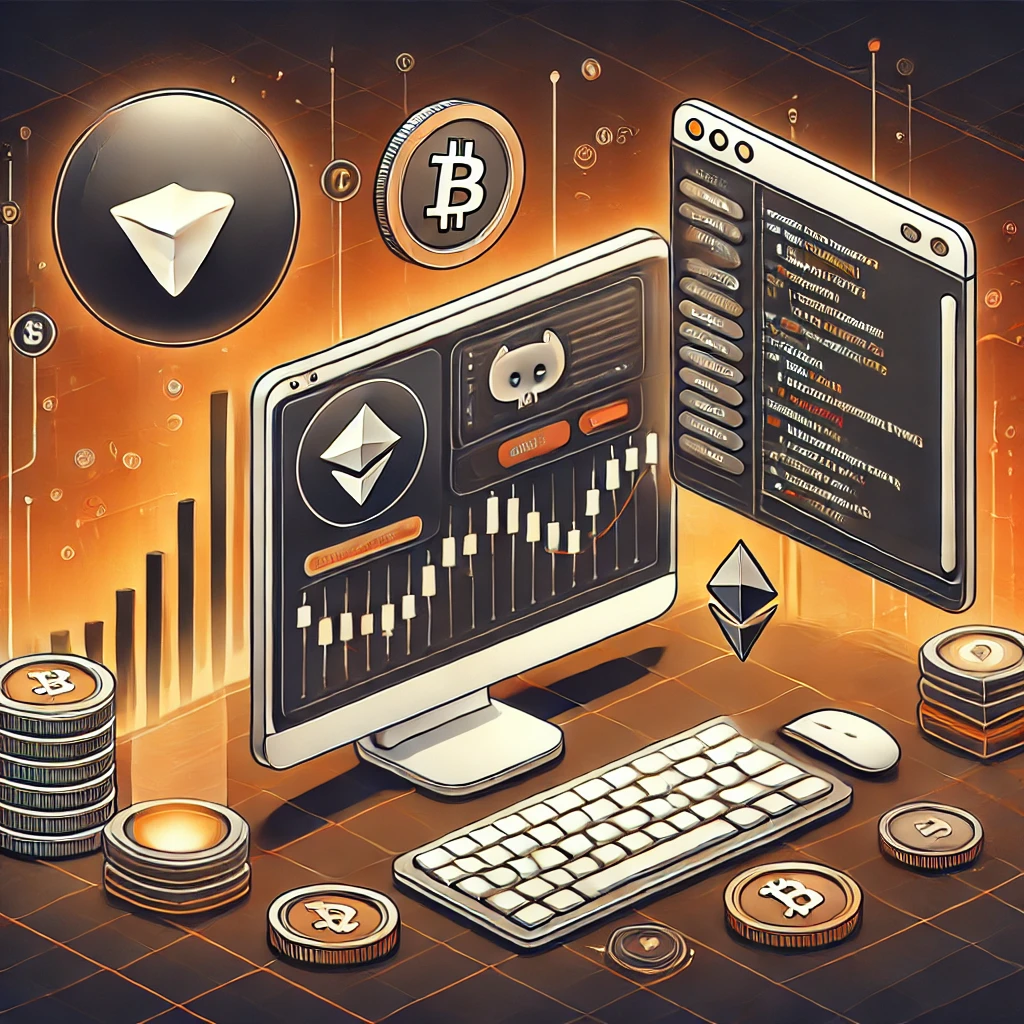
2. Creating Your Telegram Bot
If you haven’t already, create a Telegram bot using @BotFather
:
- Open Telegram and search for
@BotFather
. - Send the command
/newbot
and follow the instructions. - Save the API token provided by BotFather—this will be used to connect your script with Telegram.
Now, let's write a simple script to verify the bot’s functionality.
from telegram.ext import Updater, CommandHandler
def start(update, context):
update.message.reply_text('Hello! Your Crypto Price Alert Bot is active.')
updater = Updater('YOUR_TELEGRAM_BOT_API_TOKEN', use_context=True)
updater.dispatcher.add_handler(CommandHandler('start', start))
updater.start_polling()
updater.idle()
This script logs your bot in and responds to the /start
command.
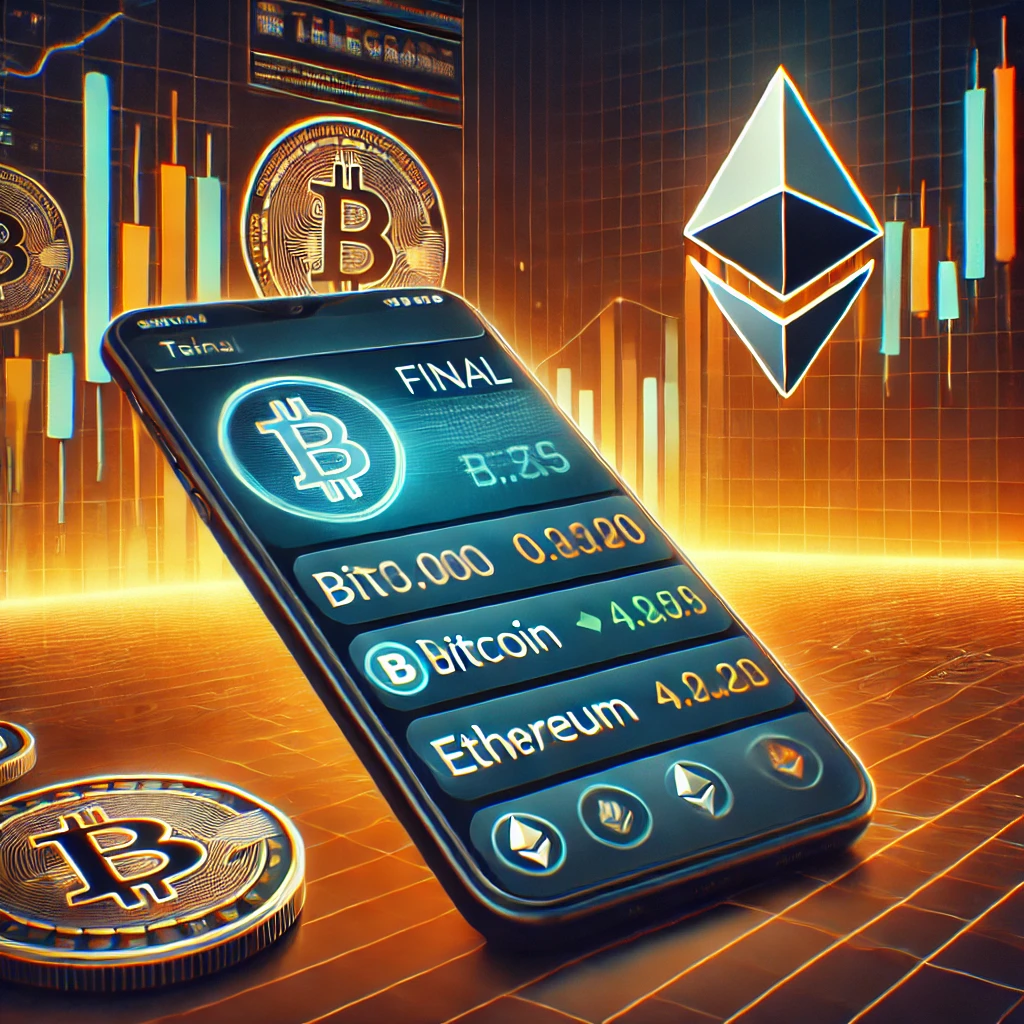
3. Integrating Cryptocurrency Data
We will now integrate cryptocurrency price data using the CoinGecko API. This API provides real-time price information for various cryptocurrencies.
Below is a sample code snippet that fetches the current price of Bitcoin:
import requests
def get_bitcoin_price():
url = 'https://api.coingecko.com/api/v3/simple/price?ids=bitcoin&vs_currencies=usd'
response = requests.get(url)
data = response.json()
return data['bitcoin']['usd']
print("Current Bitcoin Price: $", get_bitcoin_price())
You can expand this function to include more cryptocurrencies or set specific price alerts.
4. Combining Data with Telegram Alerts
Now, let's combine the cryptocurrency data with your Telegram bot to send alerts. For example, if Bitcoin’s price drops below a certain threshold, your bot will notify you.
import time
from telegram.ext import Updater, CommandHandler
# Your previously defined functions get_bitcoin_price() and start() go here
THRESHOLD_PRICE = 30000 # Set your desired price threshold
def price_alert(context):
price = get_bitcoin_price()
if price < THRESHOLD_PRICE:
context.bot.send_message(chat_id=context.job.context,
text=f"Alert! Bitcoin price has dropped to ${price}!")
def start_price_alert(update, context):
chat_id = update.message.chat_id
context.job_queue.run_repeating(price_alert, interval=60, first=0, context=chat_id)
update.message.reply_text("Price alert started. You will be notified if Bitcoin falls below $30,000.")
updater = Updater('YOUR_TELEGRAM_BOT_API_TOKEN', use_context=True)
updater.dispatcher.add_handler(CommandHandler('start', start))
updater.dispatcher.add_handler(CommandHandler('pricealert', start_price_alert))
updater.start_polling()
updater.idle()
This script checks Bitcoin’s price every 60 seconds and sends an alert to your chat if the price falls below $30,000. Customize the threshold and interval as needed.
5. Final Testing and Deployment
Before deploying your bot, run several tests to ensure:
- The bot correctly logs in and responds to commands.
- The API calls to CoinGecko are working as expected.
- The alert mechanism triggers accurately when the price crosses the threshold.
Once you’re satisfied with the functionality, deploy your bot on a server or keep it running on your PC for continuous monitoring.
Conclusion
By following this tutorial, you’ve learned how to build a cryptocurrency price alert bot on Telegram using Python. This tool can help you stay informed about market movements and make timely decisions based on real-time data.
Experiment with different cryptocurrencies, adjust alert thresholds, and expand your bot’s functionality to suit your trading or monitoring needs. Happy coding and trading!
0 Comments
Leave a Comment